Streamline Your Tests: Mocking APIs with VCR.py
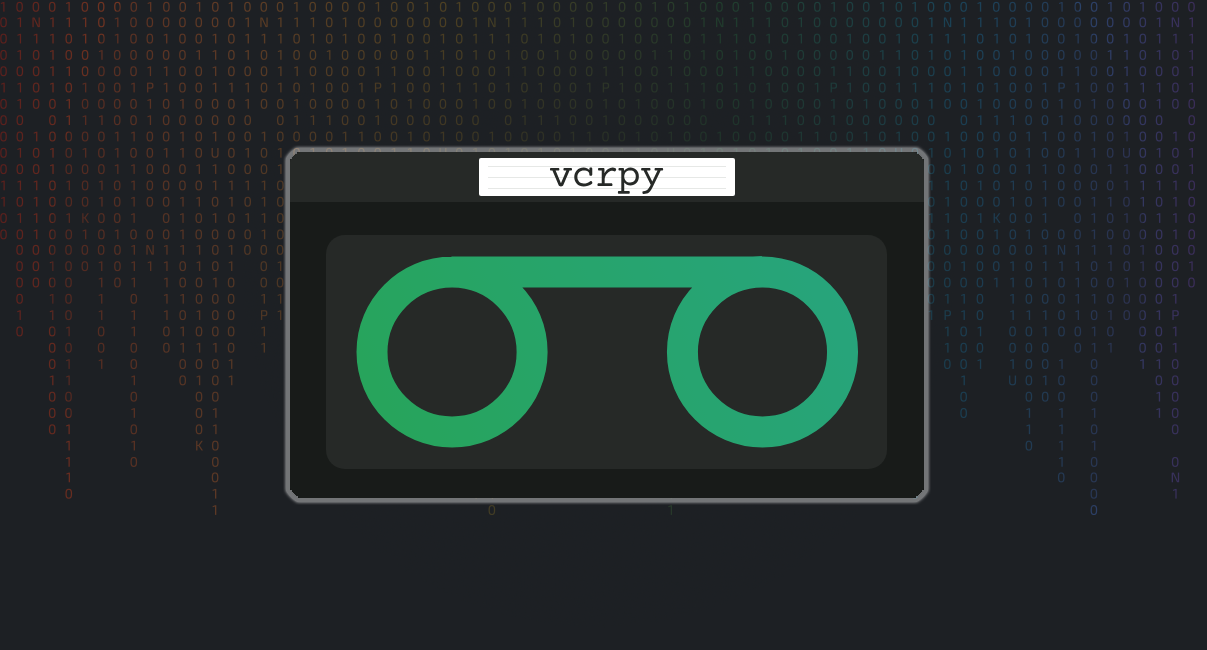
Developers typically use the unittest.mock module to patch the requests library directly, replacing real HTTP calls with mock objects. vcrpy makes it much easier.
Streamline Your Tests: Mocking APIs with VCR.py
Testing applications that interact with external services can be challenging. Third-party APIs are unpredictable; they can change, fail, or rate-limit your requests. That’s where mocking comes in handy. Traditionally, we’ve patched HTTP calls directly within our test suite, but vcrpy provides a more robust and cleaner approach because it automates some of the hard work for us.
Why do we need to test?
Testing applications is important because it’s like a safety net when you’re adding new stuff or fixing things in your application, be that a conventional application or web. Testing makes sure everything works like it’s supposed to and doesn’t mess up something else that was already fine. It’s kind of like a quality check that gives everyone involved, from the folks who build the app to the ones who use it, a big thumbs up that everything’s running smoothly. In a nutshell, good testing keeps the app reliable, and everyone using it happy and stress-free.
The Traditional Way: Patching requests
Developers typically use the unittest.mock module to patch the requests library directly, replacing real HTTP calls with mock objects. Here’s how you might typically do this:
import requests
from unittest.mock import patch, Mock
def get_user_data():
response = requests.get("https://punksecurity.co.uk/api/punks")
return response.json()
@patch('requests.get')
def test_get_user_data(mock_get):
mock_get.return_value = Mock(ok=True)
mock_get.return_value.json.return_value = {"name": "Simon Gurney", "id": "1337"}
result = get_user_data()
assert result == {"name": "Simon Gurney", "id": "1337"}
This approach works fine but can become unwieldy as the complexity of the API interactions grows. You also need to provide that API response, or at least enough of it to make the test work. For some APIs, this can become a chore in itself.
Enter VCR.py
vcrpy simplifies this process by recording HTTP interactions and replaying them for your tests. It’s like a VCR for your API calls, hence the name. You make a real request once, vcrpy records it, and then during subsequent test runs, it plays back the recorded response.
Setting up vcrpy
First, you’ll need to install vcrpy:
pip install vcrpy
Mocking with vcrpy
Here’s how to mock an API call to get user data using vcrpy:
import requests
import vcr
def get_user_data():
response = requests.get("https://punksecurity.co.uk/api/punks")
return response.json()
@vcr.use_cassette('fixtures/vcr_cassettes/punks.yaml')
def test_get_user_data_vcr():
result = get_user_data()
assert result == {"name": "Simon Gurney", "id": "1337"}
In this code, @vcr.use_cassette wraps the test function. It records the HTTP request and response to a ‘cassette’ file on the first test run. On subsequent runs, vcrpy plays back the response from this file.
Advantages of using vcrpy
- Maintainability: To update your test fixtures, you simply delete the stored cassettes.
- Reliability: Tests are not prone to failure due to API rate-limiting or downtime.
- Simplicity: Tests are easier to read and maintain.
- Quicker: You don’t need to manually capture api responses
Conclusion
Mocking API calls is an essential part of testing applications that rely on external services. While patching the requests library is a common approach, vcrpy offers a more advanced and streamlined method. By recording real HTTP interactions and replaying them, vcrpy makes your tests faster, more reliable, and a breeze to maintain. Give vcrpy a try, and you might find your tests are more like a walk in the park than a race through the wild web.
Need assistance?
If you need help building or improving your application unit or functional testing, we can help!